Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | ||||||
2 | 3 | 4 | 5 | 6 | 7 | 8 |
9 | 10 | 11 | 12 | 13 | 14 | 15 |
16 | 17 | 18 | 19 | 20 | 21 | 22 |
23 | 24 | 25 | 26 | 27 | 28 | 29 |
30 | 31 |
Tags
- npm
- 랜덤넘버
- JS
- 오버라이딩
- TypeScript
- 구글맵스
- 스프링부트
- Android
- button
- 자바스크립트
- Kotlin
- nodejs
- Linux
- array
- React
- GoogleMaps
- stylesheet
- TextView
- scrollview
- 코틀린
- 리액트
- Hook
- JavaScript
- Javscript
- Java
- fragment
- RecyclerView
- 랜덤번호
- 안드로이드
- SpringBoot
Archives
- Today
- Total
타닥타닥 개발자의 일상
JS javascript 배열 이용해서 팁 구하는 함수 만들기 / 배열 안의 숫자 평균 구하는 함수 만들기 본문
1. 10개의 가격을 포함하는 bills이라는 배열을 만들어라
Create an array 'bills' containing all 10 test bill values
const bills =[22, 295, 176, 440, 37, 105, 10, 1100, 86,52];
2. tips와 totals이라는 빈 배열을 만들어라
Create empty arrays for the tips and the totals ('tips' and 'totals')
const tips=[];
const totals=[];
3. 가격이 50이상 혹은 300 이하면 가격의 0.15배를 팁으로 하고, 아니면 가격의 0.2배를 팁으로 하는 calcTip이라는 함수를 만들어라. bills라는 배열에 담긴 모든 가격들의 팁과 팁+가격의 합을 구할수 있도록 loop를 사용해라.
Use the 'calcTip' function we wrote before (no need to repeat) to calculate tips and total values (bill + tip) for every bill value in the bills array. Use a for loop to perform the 10 calculations!
const calcTip =function(bill){
return bill >= 50 && bill <= 300 ? bill*0.15: bill*0.2;
}
for(let i =0 ; i <bills.length ; i++){
const tip = calcTip(bills[i]);
tips.push(tip);
totals.push(tip+bills[i]);
}
console.log(bills,tips,totals);
/*
//My answer
const calcTip = function(){
for(let i = 0 ; i< bills.length; i++){
tips[i] = bills[i]>=50 && bills[i]<=300 ? bills[i]*0.15 : bills[i]*0.2;
totals[i] = bills[i] + tips[i];
console.log(`bill: ${bills[i]} / tip: ${tips[i]} / total: ${totals[i]}`);
}
}
*/
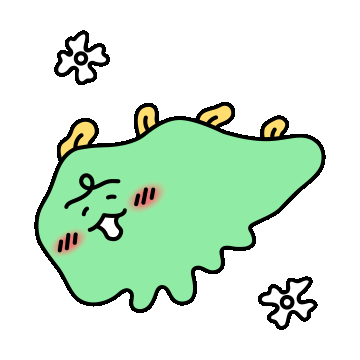
4.1. 배열에 있는 수의 평균을 구해라. 그러기 위해서는 0으로 시작하는 sum이라는 변수를 만들어야 한다. 또한 loop를 이용하여 배열의 끝까지 가야한다. 배열의 각 숫자마다 sum이라는 변수에 더해져야 한다. 이런 방법으로 배열의 끝까지 가면 모든 수를 더할수 있다.
First, you will need to add up all values in the array. To do the addition, start by creating a variable 'sum' that starts at 0. Then loop over the array using a for loop. In each iteration, add the current value to the 'sum' variable. This way, by the end of the loop, you have all values added together
const arr =[1,2,3,4,5,6,7,8,9,10];
const calcAverage = function(arr){
let sum =0;
for(let i =0; i< arr.length ; i++){
sum += arr[i];
}
return sum / arr.length;
}
console.log(calcAverage([2,3,7]));
console.log(calcAverage(totals));
console.log(calcAverage(tips));
Comments